JAVA8独有的map遍历方式(非常好用)
来源:本站原创|时间:2020-01-10|栏目:Java|点击: 次
使用JAV8 带来的map遍历方式使遍历非常简单
public class LambdaMap { private Map<String, Object> map = new HashMap<>(); @Before public void initData() { map.put("key1", "value1"); map.put("key2", "value2"); map.put("key3", "value3"); map.put("key4", 4); map.put("key5", 5); map.put("key5", 'h'); } /** * 遍历Map的方式一 * 通过Map.keySet遍历key和value */ @Test public void testErgodicWayOne() { System.out.println("---------------------Before JAVA8 ------------------------------"); for (String key : map.keySet()) { System.out.println("map.get(" + key + ") = " + map.get(key)); } System.out.println("---------------------JAVA8 ------------------------------"); map.keySet().forEach(key -> System.out.println("map.get(" + key + ") = " + map.get(key))); } /** * 遍历Map第二种 * 通过Map.entrySet使用Iterator遍历key和value */ @Test public void testErgodicWayTwo() { System.out.println("---------------------Before JAVA8 ------------------------------"); Iterator<Map.Entry<String, Object>> iterator = map.entrySet().iterator(); while (iterator.hasNext()) { Map.Entry<String, Object> entry = iterator.next(); System.out.println("key:value = " + entry.getKey() + ":" + entry.getValue()); } System.out.println("---------------------JAVA8 ------------------------------"); map.entrySet().iterator().forEachRemaining(item -> System.out.println("key:value=" + item.getKey() + ":" + item.getValue())); } /** * 遍历Map第三种 * 通过Map.entrySet遍历key和value,在大容量时推荐使用 */ @Test public void testErgodicWayThree() { System.out.println("---------------------Before JAVA8 ------------------------------"); for (Map.Entry<String, Object> entry : map.entrySet()) { System.out.println("key:value = " + entry.getKey() + ":" + entry.getValue()); } System.out.println("---------------------JAVA8 ------------------------------"); map.entrySet().forEach(entry -> System.out.println("key:value = " + entry.getKey() + ":" + entry.getValue())); } /** * 遍历Map第四种 * 通过Map.values()遍历所有的value,但不能遍历key */ @Test public void testErgodicWayFour() { System.out.println("---------------------Before JAVA8 ------------------------------"); for (Object value : map.values()) { System.out.println("map.value = " + value); } System.out.println("---------------------JAVA8 ------------------------------"); map.values().forEach(System.out::println); // 等价于map.values().forEach(value -> System.out.println(value)); } /** * 遍历Map第五种 * 通过k,v遍历,Java8独有的 */ @Test public void testErgodicWayFive() { System.out.println("---------------------Only JAVA8 ------------------------------"); map.forEach((k, v) -> System.out.println("key:value = " + k + ":" + v)); } }
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持我们。
栏 目:Java
本文地址:https://www.xiuzhanwang.com/a1/Java/8921.html
您可能感兴趣的文章
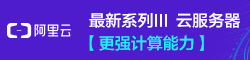
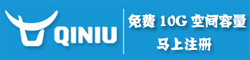
阅读排行
本栏相关
- 01-10Java实现动态模拟时钟
- 01-10Springboot中@Value的使用详解
- 01-10JavaWeb实现邮件发送功能
- 01-10利用Java实现复制Excel工作表功能
- 01-10Java实现动态数字时钟
- 01-10java基于poi导出excel透视表代码实例
- 01-10java实现液晶数字字体显示当前时间
- 01-10基于Java验证jwt token代码实例
- 01-10Java动态显示当前日期和时间
- 01-10浅谈Java中真的只有值传递么
随机阅读
- 01-11ajax实现页面的局部加载
- 01-10delphi制作wav文件的方法
- 04-02jquery与jsp,用jquery
- 01-10C#中split用法实例总结
- 08-05DEDE织梦data目录下的sessions文件夹有什
- 01-10使用C语言求解扑克牌的顺子及n个骰子
- 08-05dedecms(织梦)副栏目数量限制代码修改
- 01-11Mac OSX 打开原生自带读写NTFS功能(图文
- 08-05织梦dedecms什么时候用栏目交叉功能?
- 01-10SublimeText编译C开发环境设置