举例讲解C#编程中委托的实例化使用
合并委托
本示例演示如何创建多播委托。 委托对象的一个有用属性是:可以使用 + 运算符将多个对象分配给一个委托实例。多播委托包含已分配委托的列表。在调用多播委托时,它会按顺序调用列表中的委托。只能合并相同类型的委托。
- 运算符可用于从多播委托中移除组件委托。
using System; // Define a custom delegate that has a string parameter and returns void. delegate void CustomDel(string s); class TestClass { // Define two methods that have the same signature as CustomDel. static void Hello(string s) { System.Console.WriteLine(" Hello, {0}!", s); } static void Goodbye(string s) { System.Console.WriteLine(" Goodbye, {0}!", s); } static void Main() { // Declare instances of the custom delegate. CustomDel hiDel, byeDel, multiDel, multiMinusHiDel; // In this example, you can omit the custom delegate if you // want to and use Action<string> instead. //Action<string> hiDel, byeDel, multiDel, multiMinusHiDel; // Create the delegate object hiDel that references the // method Hello. hiDel = Hello; // Create the delegate object byeDel that references the // method Goodbye. byeDel = Goodbye; // The two delegates, hiDel and byeDel, are combined to // form multiDel. multiDel = hiDel + byeDel; // Remove hiDel from the multicast delegate, leaving byeDel, // which calls only the method Goodbye. multiMinusHiDel = multiDel - hiDel; Console.WriteLine("Invoking delegate hiDel:"); hiDel("A"); Console.WriteLine("Invoking delegate byeDel:"); byeDel("B"); Console.WriteLine("Invoking delegate multiDel:"); multiDel("C"); Console.WriteLine("Invoking delegate multiMinusHiDel:"); multiMinusHiDel("D"); } }
输出:
Invoking delegate hiDel: Hello, A! Invoking delegate byeDel: Goodbye, B! Invoking delegate multiDel: Hello, C! Goodbye, C! Invoking delegate multiMinusHiDel: Goodbye, D!
声明、实例化和使用委托
在 C# 1.0 及更高版本中,可以按以下示例所示声明委托。
// Declare a delegate. delegate void Del(string str); // Declare a method with the same signature as the delegate. static void Notify(string name) { Console.WriteLine("Notification received for: {0}", name); } // Create an instance of the delegate. Del del1 = new Del(Notify);
C# 2.0 提供了更简单的方法来编写上面的声明,如以下示例所示。
// C# 2.0 provides a simpler way to declare an instance of Del. Del del2 = Notify;
在 C# 2.0 及更高版本中,还可以使用匿名方法来声明和初始化委托,如以下示例所示。
// Instantiate Del by using an anonymous method. Del del3 = delegate(string name) { Console.WriteLine("Notification received for: {0}", name); };
在 C# 3.0 及更高版本中,还可以使用 Lambda 表达式来声明和实例化委托,如以下示例所示。
// Instantiate Del by using a lambda expression. Del del4 = name => { Console.WriteLine("Notification received for: {0}", name); };
下面的示例阐释声明、实例化和使用委托。 BookDB 类封装一个书店数据库,它维护一个书籍数据库。它公开 ProcessPaperbackBooks 方法,该方法在数据库中查找所有平装书,并对每本平装书调用一个委托。使用的 delegate 类型名为 ProcessBookDelegate。 Test 类使用该类打印平装书的书名和平均价格。
委托的使用促进了书店数据库和客户代码之间功能的良好分隔。客户代码不知道书籍的存储方式和书店代码查找平装书的方式。书店代码也不知道找到平装书后将对平装书执行什么处理。
// A set of classes for handling a bookstore: namespace Bookstore { using System.Collections; // Describes a book in the book list: public struct Book { public string Title; // Title of the book. public string Author; // Author of the book. public decimal Price; // Price of the book. public bool Paperback; // Is it paperback? public Book(string title, string author, decimal price, bool paperBack) { Title = title; Author = author; Price = price; Paperback = paperBack; } } // Declare a delegate type for processing a book: public delegate void ProcessBookDelegate(Book book); // Maintains a book database. public class BookDB { // List of all books in the database: ArrayList list = new ArrayList(); // Add a book to the database: public void AddBook(string title, string author, decimal price, bool paperBack) { list.Add(new Book(title, author, price, paperBack)); } // Call a passed-in delegate on each paperback book to process it: public void ProcessPaperbackBooks(ProcessBookDelegate processBook) { foreach (Book b in list) { if (b.Paperback) // Calling the delegate: processBook(b); } } } } // Using the Bookstore classes: namespace BookTestClient { using Bookstore; // Class to total and average prices of books: class PriceTotaller { int countBooks = 0; decimal priceBooks = 0.0m; internal void AddBookToTotal(Book book) { countBooks += 1; priceBooks += book.Price; } internal decimal AveragePrice() { return priceBooks / countBooks; } } // Class to test the book database: class TestBookDB { // Print the title of the book. static void PrintTitle(Book b) { System.Console.WriteLine(" {0}", b.Title); } // Execution starts here. static void Main() { BookDB bookDB = new BookDB(); // Initialize the database with some books: AddBooks(bookDB); // Print all the titles of paperbacks: System.Console.WriteLine("Paperback Book Titles:"); // Create a new delegate object associated with the static // method Test.PrintTitle: bookDB.ProcessPaperbackBooks(PrintTitle); // Get the average price of a paperback by using // a PriceTotaller object: PriceTotaller totaller = new PriceTotaller(); // Create a new delegate object associated with the nonstatic // method AddBookToTotal on the object totaller: bookDB.ProcessPaperbackBooks(totaller.AddBookToTotal); System.Console.WriteLine("Average Paperback Book Price: ${0:#.##}", totaller.AveragePrice()); } // Initialize the book database with some test books: static void AddBooks(BookDB bookDB) { bookDB.AddBook("The C Programming Language", "Brian W. Kernighan and Dennis M. Ritchie", 19.95m, true); bookDB.AddBook("The Unicode Standard 2.0", "The Unicode Consortium", 39.95m, true); bookDB.AddBook("The MS-DOS Encyclopedia", "Ray Duncan", 129.95m, false); bookDB.AddBook("Dogbert's Clues for the Clueless", "Scott Adams", 12.00m, true); } } }
输出:
Paperback Book Titles: The C Programming Language The Unicode Standard 2.0 Dogbert's Clues for the Clueless Average Paperback Book Price: $23.97
可靠编程
声明委托。
下面的语句声明一个新的委托类型。
public delegate void ProcessBookDelegate(Book book);
每个委托类型都描述参数的数目和类型,以及它可以封装的方法的返回值类型。每当需要一组新的参数类型或新的返回值类型时,都必须声明一个新的委托类型。
实例化委托。
声明了委托类型后,必须创建委托对象并使之与特定方法关联。在上一个示例中,您通过按下面示例中的方式将 PrintTitle 方法传递到 ProcessPaperbackBooks 方法来实现这一点:
bookDB.ProcessPaperbackBooks(PrintTitle);
这将创建与静态方法 Test.PrintTitle 关联的新委托对象。类似地,对象 totaller 的非静态方法 AddBookToTotal 是按下面示例中的方式传递的:
bookDB.ProcessPaperbackBooks(totaller.AddBookToTotal);
在两个示例中,都向 ProcessPaperbackBooks 方法传递了一个新的委托对象。
委托创建后,它的关联方法就不能更改;委托对象是不可变的。
调用委托。
创建委托对象后,通常将委托对象传递给将调用该委托的其他代码。通过委托对象的名称(后面跟着要传递给委托的参数,括在括号内)调用委托对象。下面是委托调用的示例:
processBook(b);
与本例一样,可以通过使用 BeginInvoke 和 EndInvoke 方法同步或异步调用委托。
上一篇:C#实现给图片加水印的方法
栏 目:C#教程
本文标题:举例讲解C#编程中委托的实例化使用
本文地址:https://www.xiuzhanwang.com/a1/C_jiaocheng/6718.html
您可能感兴趣的文章
- 01-10C#编程实现自定义热键的方法
- 01-10C#编程获取资源文件中图片的方法
- 01-10C#日历样式的下拉式计算器实例讲解
- 01-10C#编程自学之数据类型和变量二
- 01-10C#编程自学之开篇介绍
- 01-10C#编程自学之数据类型和变量三
- 01-10C#编程自学之运算符和表达式
- 01-10C#导出网站功能实例代码讲解
- 01-10C#编程自学之类和对象
- 01-10C#编程和Visual Studio使用技巧(下)
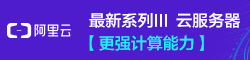
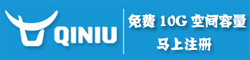
阅读排行
本栏相关
- 01-10C#通过反射获取当前工程中所有窗体并
- 01-10关于ASP网页无法打开的解决方案
- 01-10WinForm限制窗体不能移到屏幕外的方法
- 01-10WinForm绘制圆角的方法
- 01-10C#实现txt定位指定行完整实例
- 01-10WinForm实现仿视频播放器左下角滚动新
- 01-10C#停止线程的方法
- 01-10C#实现清空回收站的方法
- 01-10C#通过重写Panel改变边框颜色与宽度的
- 01-10C#实现读取注册表监控当前操作系统已
随机阅读
- 01-10使用C语言求解扑克牌的顺子及n个骰子
- 01-10C#中split用法实例总结
- 01-11ajax实现页面的局部加载
- 04-02jquery与jsp,用jquery
- 01-11Mac OSX 打开原生自带读写NTFS功能(图文
- 08-05dedecms(织梦)副栏目数量限制代码修改
- 08-05DEDE织梦data目录下的sessions文件夹有什
- 08-05织梦dedecms什么时候用栏目交叉功能?
- 01-10SublimeText编译C开发环境设置
- 01-10delphi制作wav文件的方法